react-svg-donuts
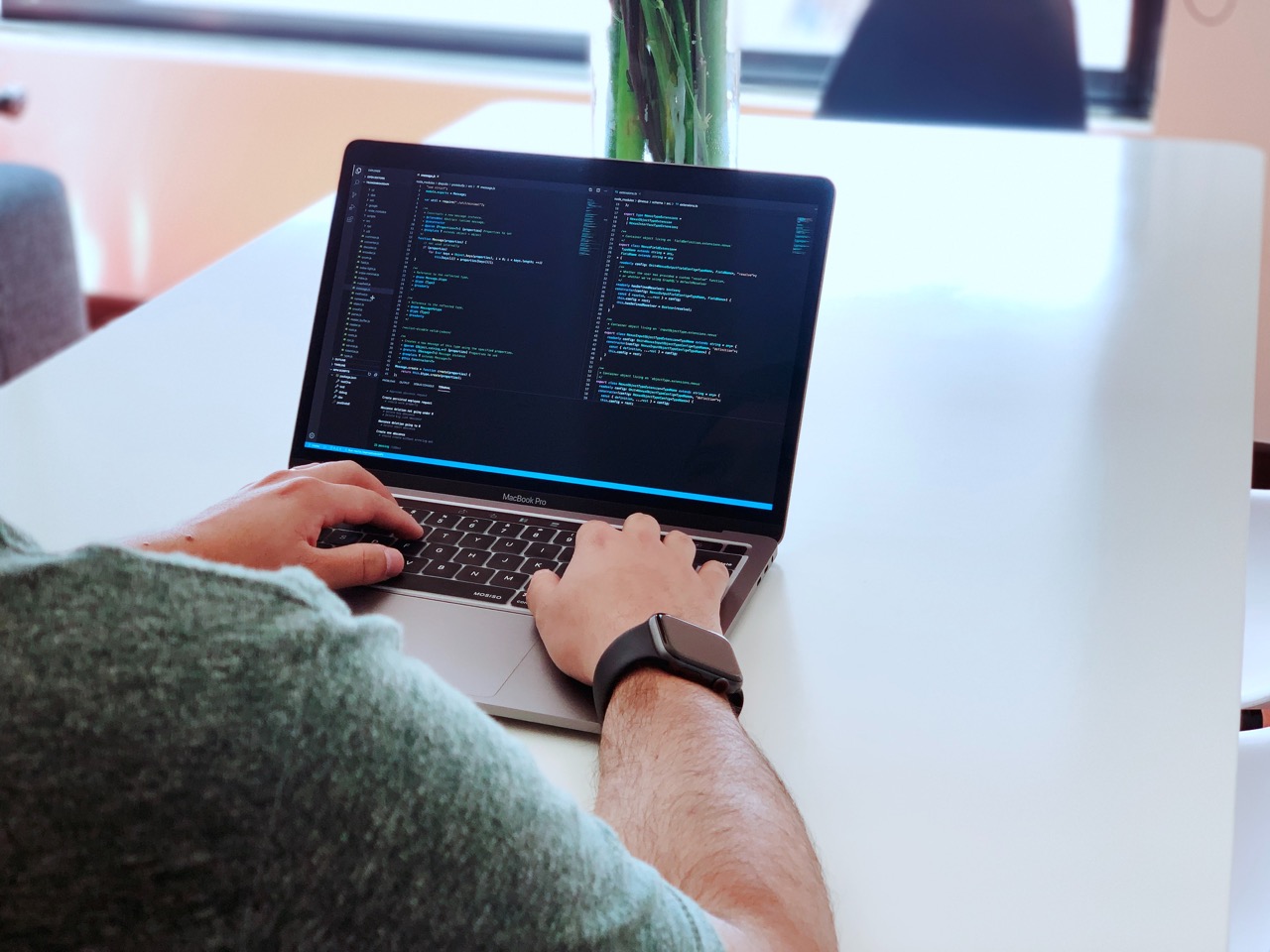
React SVG Donuts
A React component for simple (and complex) SVG donuts.
The current version depends on the Hooks API introduced with React 16.8. If you need legacy React support, please use a 1.x.x version.
Visitor stats
Code stats
Demo
TL;DR here is the demo
Dependencies
- NodeJS
- NPM / Yarn
- React and ReactDOM
- A ReactJS application
Usage
First install the package
$ npm i react-svg-donuts
# or
$ yarn add react-svg-donuts
Then
import React from 'react';
import { Donut, ComplexDonut } from 'react-svg-donuts';
// The donut will be half filled
const progress = 50;
// The value will be wrapped inside a <strong> tag.
const renderProgress = progress => <strong>{progress}%</strong>;
const MyComponent = () => (
<>
<Donut progress={progress} onRender={renderProgress} />, document.getElementById('demo')
<ComplexDonut
size={200}
parts={[
{
color: '#FF8A80',
value: 230
},
{
color: '#FF80AB',
value: 308
},
{
color: '#B9F6CA',
value: 520
},
{
color: '#B388FF',
value: 130
},
{
color: '#8C9EFF',
value: 200
}
]}
radius={80}
thickness={40}
startAngle={-90}
/>
</>
);
Props
Donut props
Prop | Type | Required | Default | Description |
---|---|---|---|---|
prefix |
string |
false | 'donut' | String used as a prefix for the CSS class names |
progress |
number |
false | 0 | A number between 0 and 100 |
onRender |
Function |
false | (progress) => {progress} | Function which runs after the Donut is rendered and returns a ReactNode |
Complex donut props
Prop | Type | Required | Default | Description |
---|---|---|---|---|
size | number | false | 160 | The width and height of the donut |
parts | { color: string; value: number; }[] | false | [] | The donut parts |
radius | number | false | 60 | The radius of the |
className | string | false | '' | Custom CSS class name for the Donut |
thickness | number | false | 30 | Stroke width of the |
textProps | SVGProps for SVGTextElement | false | {} | Additional props for the |
startAngle | number | false | -90 | Number between -360 and 360 |
circleProps | SVGProps for SVGCircleElement | false | {} | Additional props for the |
CSS
There is a predefined stylesheet which can be used as is. If you want it, just import it:
@import 'react-svg-donuts/src/index.css';
or
import 'react-svg-donuts/src/index.css';
LICENSE
MIT
Connect with me: